Display List
- Create task list component
Build a component to display the list of tasks.
- Use Angular directives
Master ngFor and other directives for list rendering.
- Style the list
Apply styling to make the task list visually appealing.
HTML Structure
To display an array of elements, the expected HTML structure is:
<table class="table"> <thead> <tr> <th>Name</th> <th>Date</th> <th>Actions</th> </tr> </thead> <tbody> <tr> <td>Follow the course</td> <td>05/24/2024</td> <td></td> </tr> <tr> <td>Follow the course</td> <td>05/24/2024</td> <td></td> </tr> </tbody></table>
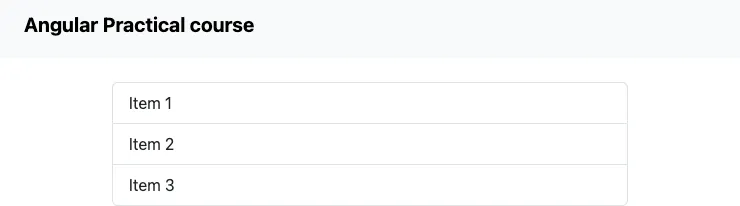
However, what happens if you want to display a dynamic array of elements that changes over time? What if a new task is added?
You’ll want to rely on the tasks variable to display the correct list of tasks. If the tasks are updated, the view (HTML Template) should be updated as well.
Angular Control Flow
Introduced in Angular v17 and stable since v18, the new Control flow feature is the modern way in Angular to define rules for rendering HTML dynamically.
It somehow replicates the Javascript behavior at using if
, for
loops and switch
statements in your templates.
Like in Javascript, we want some content to be either:
- displayed based on a condition (if)
- iterated over with a loop (for)
- displayed if matching a certain criteria (switch)
For the current lesson, we will use @for
in the HTML template to iterate over the list of tasks.
It’s made of 2 parts:
- the
@for
keyword with a definition of the list to iterate over and some options - a block of code to execute for each item in the list
// Loop Definition@for (task of tasks; track task.id) {
// Loop Body<tr> <td>{{ task.title }}</td> <td>{{ task.createdAt }}</td> <td></td></tr>
}
Loop definition
Like in Javascript we need to define 2 things:
- the list to iterate over
- a reference to use the value of each list item (you can name it whatever you want)
In our case, we want to iterate over the list of tasks we defined in our component.ts
file and use the value of each task in the loop.
The expected format is <reference> of <list>
.
@for (task of tasks)
Angular also expects a track
option to help it optimize the rendering of the list.
In our case, we will use the id
of the task to track it.
@for (task of tasks; track task.id)
If any rerendering of the list is needed, Angular will use the id
of the task to determine if it’s the same task or a new one.
If that’s the same, Angular will reuse the existing DOM element rather than creating a new one.
Loop body
The loop body is the code that will be executed for each item in the list.
Like in Javascript, we use {}
to define the body of the loop.
@for (task of tasks; track task.id) {<tr> <td>Follow the course</td> <td>05/24/2024</td> <td></td></tr>}
Signals value
Rather than defining a simple property, we made it a Signal
ealier: to get the value of a Signal, we need to call it with ()
, like tasks()
.
@for (task of tasks(); track task.id) {<tr> <td>{{ task.title }}</td> <td>{{ task.createdAt }}</td> <td></td></tr>}
Let’s now use this in our application.
Instructions
-
Update the
src/app/task-list.html
file.task-list.html <table class="table"><thead><tr><th scope="col">Name</th><th scope="col">Date</th><th scope="col">Actions</th></tr></thead><tbody>@for (task of tasks(); track task.id) {<tr><td>Follow the course</td><td>05/24/2024</td><td></td></tr>}</tbody></table> -
Go back to your browser and check the following page:
Your list is now displayed in the browser.
You should see Task
repeated as many times (2) as there are tasks in the list.
In this chapter, you discovered one feature from the Angular control flow, to iterate over a list of elements and display them in the HTML template. That’s a quite common task as most of data used in applications is dynamic and changes over time.
Official Angular Documentation: @for